Due at 11:59pm, Wednesday, March 9, 2016
by uploading submission to Stellar.
You must complete the self-assessment form before you hand in the assignment.
You should make sure that your problem set renders correctly on Chrome (which we'll be using for evaluating your projects) and in either Firefox or Safari.
This assignment explores the following topics related to GUI output:
- the object approach;
- the stroke approach (also called vector graphics);
- the pixel approach;
- handling events sent from a model to a view.
Overview
In this problem set, you will implement a browser-based view of Checkers, a two-player game. You don't have to implement the rules, since we provide an implemented model that includes the rules as methods you can call. But in case you haven't played the game before, the rules are summarized as follows:
- Each player starts with their pieces lined up on the two rows closest to their own side.
- Players play from opposite ends of the board, and take turns moving pieces diagonally-forward from one square to another.
- A turn consists of moving one piece diagonally-forward to an adjacent unoccupied square.
- If one player's piece, the opponent's piece, and an empty square are lined up, then the first player can "jump" the other player's piece. In this case, the first player jumps over the other player's piece onto the empty square and takes the other player's piece off the board. Multiple sequential jumps by one piece are allowed on a single turn.
- If a player's piece moves into the last row on the opposite side, it becomes a king piece, and can now move forward or backward.
- The game ends when a player loses all of their pieces, or is put in a position where they cannot move.
You'll implement using a mix of component, stroke, and pixel techniques. In this problem set, you'll only be concerned with output. In the next problem set, you'll add input handling to your views, so that a user can click and drag checkers using the mouse.
You may need to use HTML5 Canvas on this assignment, and these references may be helpful:
- HTML5 Canvas: Introduction
- HTML5 Canvas: Draw Lines and Filled Shapes
- HTML5 Canvas: Animation Basics
- Mozilla Canvas Tutorial
If you want to review HTML/CSS/Javascript/jQuery/jQueryUI, here are useful video tutorials picked by the course staff:
- HTML Tables and Layouts
- HTML Forms
- Introduction to Styling with CSS
- CSS Absolute and Relative Positioning Tutorial
- Making Divs Side by Side Using CSS
- jQuery - Introduction to Selectors
- jQuery - ID Selector
- jQuery - Click Event Handler
- jQuery - addClass()
- jQuery UI Datepicker
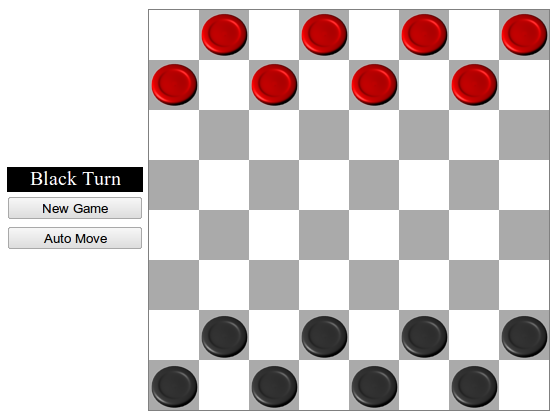
Provided Resources
We provide you with a lot of existing code for this assignment. You can get it all at once here:
- ps2.zip: a zip file containing a main page, stylesheet and javascript libraries for this problem set.
In the root folder of the project are:
- the following files:
- board.js: a javascript file containing the Board class
- boardEvent.js: a javascript file containing the BoardEvent class
- checker.js: a javascript file containing the Checker class
- index.html:: a skeleton file for your user interface
- mainLayout.css: a stylesheet file for index.html
- rules.js: a javascript file containing classes for Checker game rules
- the following folders:
- graphics: a folder containing all the graphics files
- external_js: a folder for external JavaScript libraries (contains jQuery)
The javascript board model actually has pieces on it, but you won't see them until you've implemented the pieces display.
Problem 1: Board (30%)
Fill in the skeleton of index.html so that it displays a 400x400-pixel
checkerboard. The upper left square should be white. The number of squares
across the board should be dynamically determined by the BOARD_SIZE
variable in the
code. This size defaults to 8x8, but you can change it to any value n by adding ?size=n
to
the end of the URL, e.g. index.html?size=16
. No matter how many squares are in the
checkerboard, it should always be 400x400 pixels. Don't worry too much about odd board sizes.
You can use either canvas (the stroke approach) or HTML elements (the object approach) to solve this problem.
Problem 2: Checkers (40%)
Display all the checkers on the board using HTML elements (the object approach). Four pictures are provided for you (red-piece.png, black-piece.png, red-king.png, and black-king.png, found in the graphics folder). Please don't replace them with different pictures.
Be sure that as the board scales to different sizes, so do the checkers. They should also be centered on their respective squares regardless of board size.
Your view must update when the board changes so that it displays the current state of the board at all times. You can test this by clicking on Auto Move, which produces legal board moves in the model, and New Game.
Black and red should take turns to make a move. As such, you will need to update and keep track of whose turn it is to move. Add a visual cue regarding the respective turns to move above the buttons. Ensure you update the text (e.g. from "Black Turn" to "Red Turn") and background color of the visual cue based on whose turn it is. Remember, black moves first.
Problem 3: Move Feedback (30%)
When a checker moves from one place to another, draw a yellow arrow from the center of its old square to the center of its new square, appearing above all checkers. In the case of a double jump, you only need to draw one arrow from the starting square to the ending square. This arrow should persist until the next change to the board. If you are having problems thinking about how to draw an arrow, take a look at Exercise 4 of the Javascript/jQuery tutorial
Going Further
If you found this assignment easy and you're inclined to go further, try the following:
- Animate checkers when they move
- Draw the checkerboard in perspective, so that it's more obvious to the user which side they're playing.
What to Hand In
You must complete the self-assessment form before you hand in the assignment.
Package your completed assignment as a zip file that contains all of your files (including all necessary external libraries). Failure to include all necessary files for running your program will result in a loss of points from your final grade. (Please test by unzipping your zip file into, say, your /tmp directory, turning off your Internet connection, and then opening your page to see if it still works.)
List your collaborators in the comment at the top of index.html, or explicitly say that you discussed the assignment with nobody. Collaborators are any people you discussed this assignment with. This is an individual assignment, so be aware of the course's collaboration policy.
Here's a checklist of things you should confirm before you hand in:
- Complete the self assessment.
- Make sure your collaborators are named in index.html.
- Make sure that the page renders correctly on Chrome (which we'll be using for evaluating your projects) and in either Firefox or Safari.
- Make a fresh folder and unpack your zip file into it.
- Make sure all assets (images, jQuery source, JS files, CSS files, etc.) used by your code are found in the fresh folder and load successfully.
Submit your zip file on Stellar.