This chapter discuses the design and implentation of the interactive user experience, including all dynamical aspects of the project. A complete description of the design of the eletronics and embedded software is provided. We first describe the gameplay, and then we discuss how we designed the system that makes that gameplay possible.
Gameplay
When the players enter the room, they hear ancient Egyptian music playing in the background, and they see a locked sarcophagus in the center of the room. The goal of the players is find a way to unlock and open the sarcophagus by solving a 3-level challenge puzzle, which requires that players be interacting with both the exterior of the sarcophagus and with the room walls in a particular way. The sarcophagus is decorated in various hand-sized hieroglyphs, of which exactly 9 are blinking white. The sarcophagus also features 3 scarabs, which clearly stand out from the rest of the decorations, as well as a scoreboard that indicates the three levels that need to be solved.
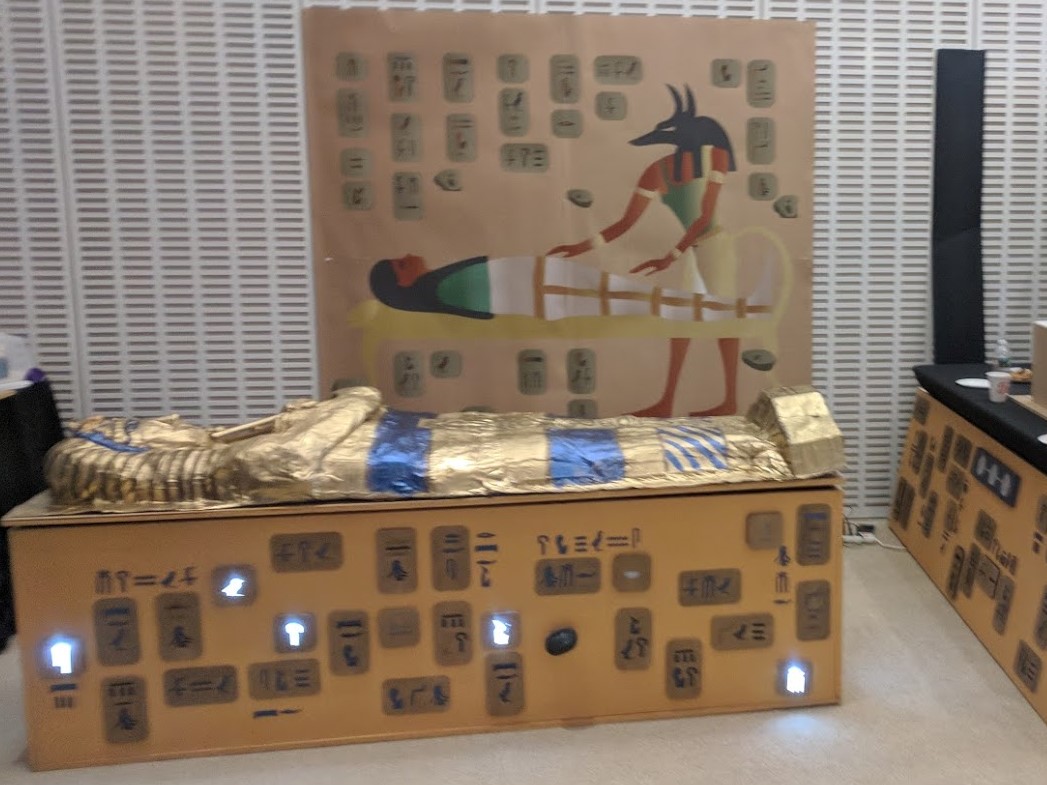 |
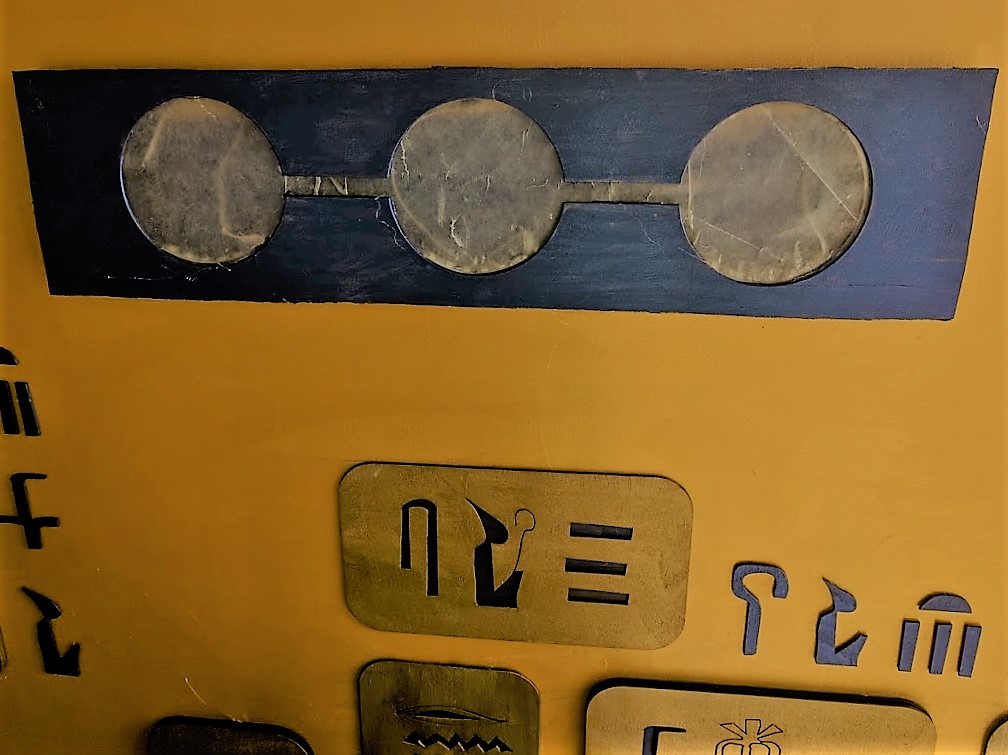 |
The walls of the room also have the same 9 hieroglyphs, which are touch sensitive buttons. However, pressing any or all of the hieroglyph-buttons on the wall causes nothing to change, yet. The players notice the 3 scarabs / beetles on the sarcophagus, and they decide to touch them simultaneously. At this point, the 9 hieroglyphs on the sarcophagus stop blinking, and only 3 remain permanently lit. The players now have to go search for those same 3 hieroglyphs on the walls of the room and press them simultaneously. Upon doing so, the players hear an unlocking sound, and they see that level one of the scoreboard is now lit (indicating completion of that level). Now they are in the next level. The background music is now playing at a faster tempo, and the 9 LEDs on the sarcophagus are flashing again - but at higher frequency. To solve this level, the players have to repeat the same process. They press the 3 scarabs on the sarcophagus simultaneously, and now 3 different LEDs remain permanently lit. The players have to find the corresponding 3 hieroglyphs on the walls and press them simultaneously. And now, the scoreboard shows that level 2 is solved. In addition, the sarcophagus lid ripples slighly - indicating visually and auditorily that it is almost ready to be opened. One more level remains. The background music is now playing at an even faster tempo, and the 9 hieroglyphs are flashing red at an even faster rate. The players repeat the same actions of pressing the scarabs simultaneously, seeing which hieroglyphs remain lit, and then pressing the 3 hieroglyphs on the wall simultaneously. The final level is now solved. The background music stops. The hieroglyphs are now all lit. After a short delay, the lid of the sarcophagus starts opening slowly with a creaking sound. Upon glancing inside, the players see a dark bottomless abyss, which is achieved by an infinity mirror effect.
Implementation
After consulting with Dr. Wallace about the scope of this project, we were advised to simplify the implementation and focus our efforts on making the sarcophagus fully interactive, and make only a looks-like implementation for the walls. This made our project much more manageable to implement, because otherwise, we would have needed two completely separate subsystems - one for the sarcophagus and another for the walls - and additionally a wireless communication network between them. By focusing our system-design efforts only on the sarcophagus, we were able to complete all aspects of the implementation just in time for the final presentations.
Electronics Design
We had 9 LEDs (tiles) and 3 buttons (scarabs) on the sarcophagus exterior. And we needed a subset of 3 LEDs to become active when the 3 buttons are pressed simultaneously - where a different subset of 3 LEDs becomes active each time. We thus grouped the 9 LEDs into 3 groups of 3 LEDs, where each group is controlled by one MOSFET. Additionally, we had 3 more LEDs for the scoreboard, where each had to be controlled individually via its own MOSFET. As for the 3 buttons, we used the microcontroller’s internal pullup resistors, thus one end of a button was connected to an MCU pin and another end to GND. When the button is not pressed, the MCU pin would be at logic HIGH via the internal pullup resistor, and pin would go to logic LOW. Thus, each button was implemented as an active low configuration. To control the background music, we bought a cheap MP3/MP4 player from Amazon, that we hacked and wired up to the Microcontroller - where instead of pressing the buttons on the device itself, we could control the buttons by applying the appropriate logic levels on the MCU’s pins connected to those buttons. To control the linear actuator, we initially used Adafruit DRV8871 DC motor driver. To know the exact position of the actuator, we used the internal 10K potentiometer inside the actuator, which we wired to one of the analog input pins on our microcontroller. We designed a printed circuit board in Eagle based on the Teensy 3.5 microcontroller.
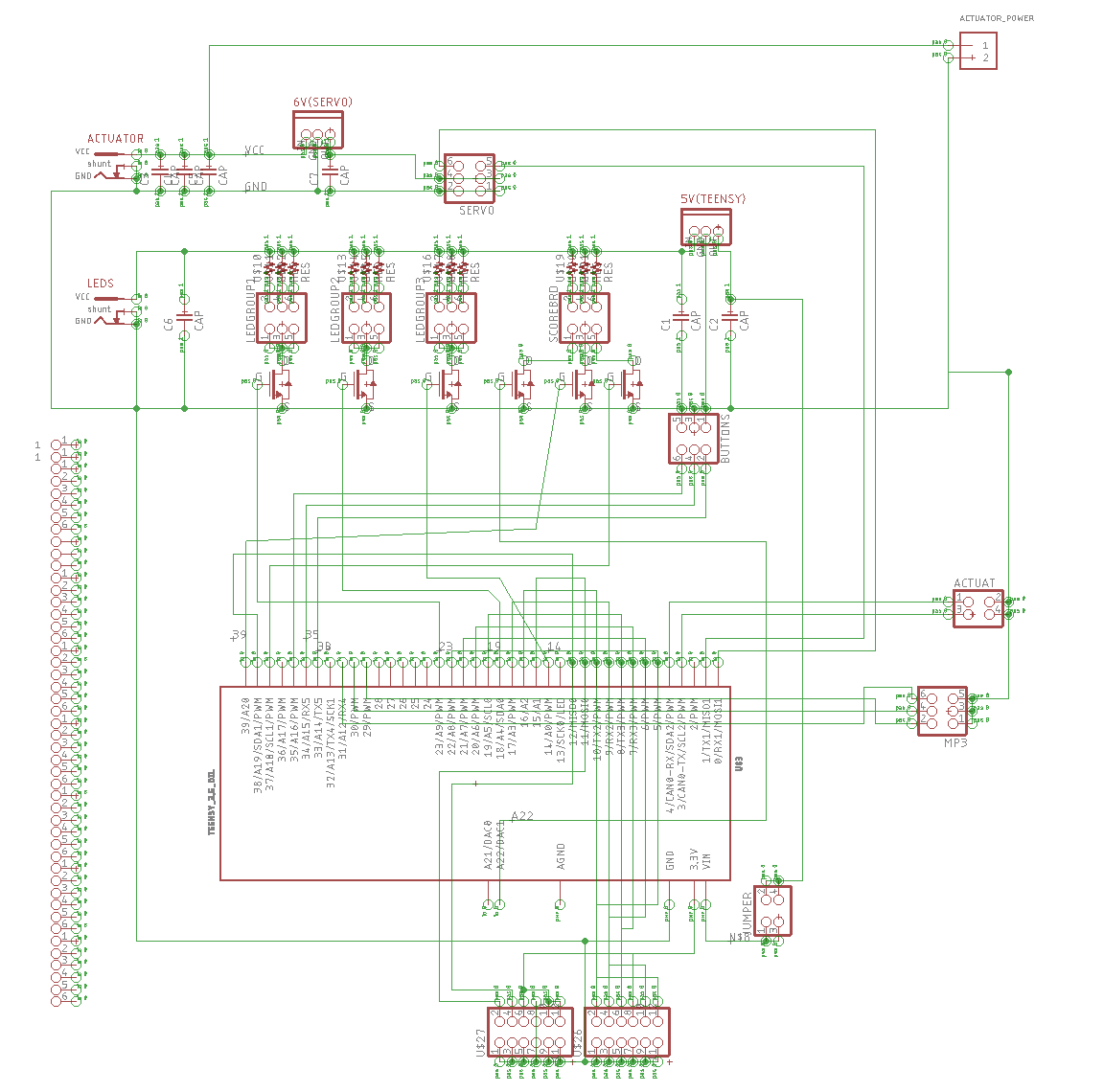 |
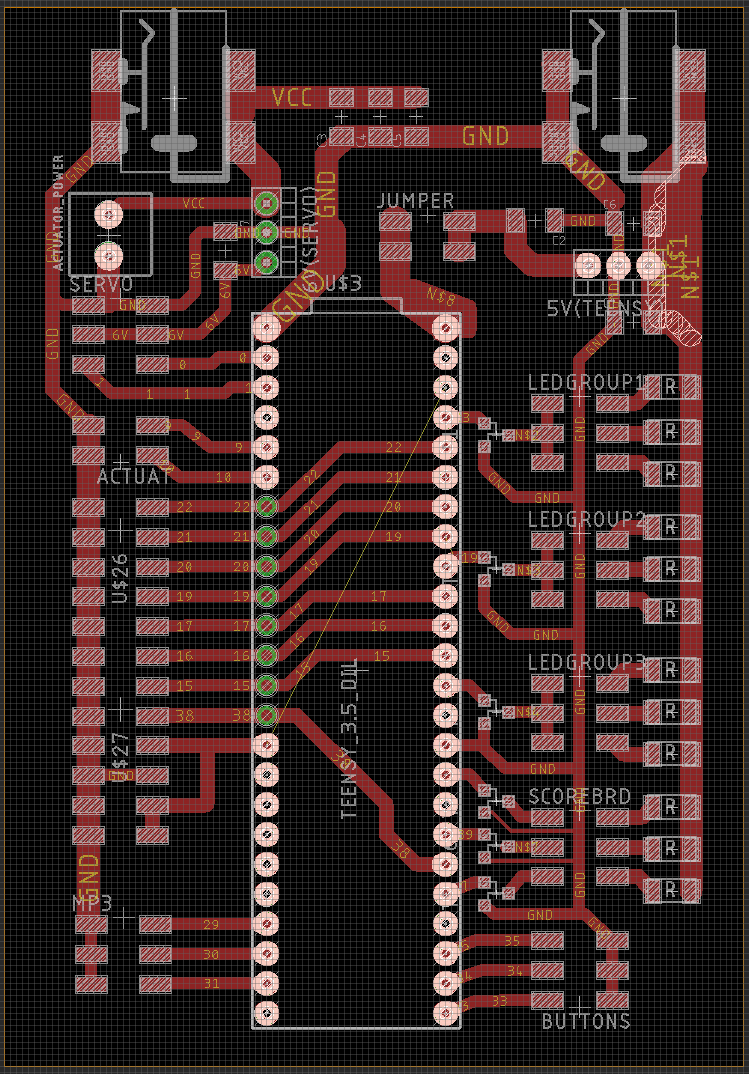 |
After fabricating the board and soldering all of the components, however, we found out that that the 3.3V on the GPIO pins on the teensy is insufficient to change the state of the MOSFETS we had available, which required 4.5V. Moreover, we found that our DC Motor driver was not capable of supplying the amount of current necessary for the linear actuator to open the lid of the sarcophagus. We had spent more than 2 days designing and fabricating the driving electronics, and by the time we found out that they were not powerful enough to drive the interactive experience, we had less than 20 until the final presentations. Making another circuit board for a 5V microcontroller was not going to be possible given the time constraint, and we did not even have enough components for another circuit board. Nevertheless, we found a way out of this difficult situation, by realizing that we could use a relay board instead of MOSFETS to drive the LEDs. Furthermore, we also realized that we could create the equivalent of a High-Current H-Bridge for powering the linear actuator by using another relay board with 4 relays. The picture below shows the final implementation based on the relay approach. The relay board on the left is for controlling the LEDs, while the one on the right is wired up as an H-Bridge for providing bidirectional control to the linear actuator.
 |
To connect the LEDs to the relays and to power, a PCB was designed and fabricated that provides easy interconnects to both ribbon cables for the LEDs and jumper cables for the MCU.
A more detailed view of how the 4-relay board was wired up as an H-Bridge is presented on the following image. The red and the black terminals, which are seen being connected to the 1st and 2nd relays are wires of the linear actuator. The input terminals of the relay are nonnected to GPIO pins of the microcontroller. This particular relay board is active low, thus when LOW signal is applied to an input pin, that is when the relay closes.
Embedded Software
To implement the dynamical behavior of the system, we first encapsulated each behavioral component into its own separate function. Essentially, we created an API containing the functions listed below
fullyOpenLid()
- retracts the linear actuator until the internal potentiometer reaches 873.
fullyCloseLid()
- expands the linear actuator until the internal potentiometer reaches 690.
blinkAllLeds(ms)
- blink al 9 LEDs without at a rate specified by the argument of the function. Implemented as nonblocking.
ledGroup1On23Off()
- keep group 1 LEDs on, and groups 2 and 3 off.
ledGroup2On13Off()
- keep group 2 LEDs on, and groups 1 and 3 off.
ledGroup3On12Off()
- keep group 3 LEDs on, and groups 1 and 2 off.
allLedGroupsOn()
- keep all 9 LEDs on.
playExperience1()
- execute everything that needs to happen when level 1 is complete.
playExperience2()
- execute everything that needs to happen when level 2 is complete.
playExperience3()
- execute everything that needs to happen when level 3 is complete.
The entire code that makes this interactive user experience possible, including all the function definitions, is copied below.
#define ledGroup1Pin 13 //controls 3 leds all connected to the same pin
#define ledGroup2Pin 12 //controls 3 leds
#define ledGroup3Pin 11 //controls 3 leds
#define scarab1Pin 7
#define scarab2Pin 6
#define scarab3Pin 5
#define actuatorIn1 3
#define actuatorIn2 4
#define relay1 A5
#define relay2 A4
#define relay3 A3
#define relay4 A2
#define potentiometerPin A1
#define masterBtnPin 2
unsigned long prevMillis = 0; // will store last time LED was updated
bool LEDState = 1; //OFF, because active low
bool reset = 0;
bool blinkState = true; //blinking flag
int btnPressCount=0;
int btnReleaseCount=0;
bool masterBtnHasBeenPressed=false; //master button to advance the level.
bool scarabHasBeenPressed=false;
bool experience1Complete=false;
bool experience2Complete=false;
bool experience3Complete=false;
bool experience4Complete=false;
int level=0;
void setup() {
pinMode(ledGroup1Pin, OUTPUT);
pinMode(ledGroup2Pin, OUTPUT);
pinMode(ledGroup3Pin, OUTPUT);
pinMode(scarab1Pin, INPUT_PULLUP);
pinMode(scarab2Pin, INPUT_PULLUP);
pinMode(scarab3Pin, INPUT_PULLUP);
pinMode(actuatorIn1,OUTPUT);
pinMode(actuatorIn2,OUTPUT);
pinMode(relay1,OUTPUT);
pinMode(relay2,OUTPUT);
pinMode(relay3,OUTPUT);
pinMode(relay4,OUTPUT);
digitalWrite(relay1,HIGH);
digitalWrite(relay2,HIGH);
digitalWrite(relay3,HIGH);
digitalWrite(relay4,HIGH);
pinMode(potentiometerPin, INPUT);
pinMode(masterBtnPin, INPUT_PULLUP);
}
void loop() {
//1. The LEDs are blinking when the user enters the room.
//2. They have to touch the 3 beetles at the same time to get only one LED group lit.
// a. set blinkState=false
//3. We press the master button to advance the level.
// a. set blinkState=true
//4. The LEDs are blinking again.
//5. The users press the 3 beatles.
//6. We press the master button.
// if(reset==true){
// blinkState=true;
// level=0;
// }
//(1) LEDs are blinking.
if(blinkState==true){
blinkAllLeds(300);
//moveActuatorDown(500);
//fullyOpenLid();
//fullyCloseLid();
//delay(1000);
}
else if(blinkState==false){
if(level==0) ledGroup1On23Off();
else if(level==1) ledGroup2On13Off();
else if(level==2) ledGroup3On12Off();
}
//if all 3 scarabs are pressed, then set the blink flag to stop blinking
if(digitalRead(scarab1Pin)==0 && digitalRead(scarab2Pin)==0 && digitalRead(scarab3Pin)==0){ //when all pressed
blinkState=false;
}
//Users now have to find the corresponding glyphs on the wall and press them. For the demo, we will press a master button,
//and then level1 will be unlocked. Sercophagus will do something.
//MASTER BUTTON REPLACES THE FUNCTIONALITY OF THE INTERACTIVE WALL:
//I want to increment the btnCount when the button is pressed and released.
if(masterBtnHasBeenPressed==false && digitalRead(masterBtnPin) == 0){ //if btn has not been pressed before AND is now being pressed
masterBtnHasBeenPressed=true;
btnPressCount++; //register the pressing when the button is pressed.
if(btnPressCount==5) btnPressCount=0; //reset the button press count in case the button ahs been pressed 5 times.
}
if(masterBtnHasBeenPressed==true && digitalRead(masterBtnPin)==1){ //if btn has been pressed nefore AND is not currently released
masterBtnHasBeenPressed=false; //reset the flag
btnReleaseCount++; //register the relese when the button is released. (we don't need this)
if(btnReleaseCount==5) btnReleaseCount=0;
}
if(btnReleaseCount==1 && experience1Complete==false){ //move to the next level
experience1Complete=true;
playExperience1();
//The effect of the next 2 is on the 2nd if-block.
blinkState=true; //start blinking all LEDs again
level=1; //set the level to 1
}
else if(btnReleaseCount==2 && experience2Complete==false){
experience2Complete=true;
playExperience2();
//The effect of the next 2 is on the 2nd if-block.
blinkState=true; //start blinking all LEDs again
level=2; //set the level to 2. The effect of this
}
//Before we press the button for a third time, the last group of LEDs is ON, and so the blink state is false.
//When players touch the wall for the final time, we press the master button, and then the entire coffin lits up.
else if(btnReleaseCount==3 && experience3Complete==false){
experience3Complete=true;
playExperience3();
level=3; //this is unnecessary, but can be used as a reset.
fullyOpenLid();
}
else if(btnReleaseCount==4 && experience4Complete==false){
experience4Complete=true;
blinkState=true;
level=0;
fullyCloseLid();
btnReleaseCount=0;
}
//When we change the leve to level 3, what should happen then?
//now I need to write what happens for the next 2 levels to the LEDs on the sercophagus.
}
void openLid(int milliseconds){ //use a while loop where the condition is the value on the pin.
//run for the specified number of milliseconds
int currenttime=millis();
while(millis()-currenttime < milliseconds){
digitalWrite(relay1,LOW);
digitalWrite(relay4,LOW);
}
//enter break mode
digitalWrite(relay2,HIGH);
digitalWrite(relay3,HIGH);
digitalWrite(relay1,HIGH);
digitalWrite(relay4,HIGH);
}
void closeLid(int milliseconds){ //speed is from 0 to 255
//run for the specified number of milliseconds
int currenttime=millis();
while(millis()-currenttime < milliseconds){
digitalWrite(relay2,LOW);
digitalWrite(relay3,LOW);
}
//enter break mode
digitalWrite(relay2,HIGH);
digitalWrite(relay3,HIGH);
digitalWrite(relay1,HIGH);
digitalWrite(relay4,HIGH);
}
void blinkAllLeds(int interval){ //the argument is the time between blinks
if(millis()-prevMillis >= interval){
prevMillis=millis();
LEDState=!LEDState;
digitalWrite(ledGroup1Pin,LEDState);
digitalWrite(ledGroup2Pin,LEDState);
digitalWrite(ledGroup3Pin,LEDState);
}
}
void ledGroup1On23Off(){
digitalWrite(ledGroup1Pin,LOW);
digitalWrite(ledGroup2Pin,HIGH);
digitalWrite(ledGroup3Pin,HIGH);
}
void ledGroup2On13Off(){
digitalWrite(ledGroup1Pin,HIGH);
digitalWrite(ledGroup2Pin,LOW);
digitalWrite(ledGroup3Pin,HIGH);
}
void ledGroup3On12Off(){
digitalWrite(ledGroup1Pin,HIGH);
digitalWrite(ledGroup2Pin,HIGH);
digitalWrite(ledGroup3Pin,LOW);
}
void allLedGroupsOn(){
digitalWrite(ledGroup1Pin,LOW);
digitalWrite(ledGroup2Pin,LOW);
digitalWrite(ledGroup3Pin,LOW);
}
void playExperience1(){
//playSound();
}
void playExperience2(){
//lidRipple();
//fanOn();
}
void playExperience3(){
allLedGroupsOn(); //turn all LEDS on the coffin on.
//sarcophagusOpen();
//infinityMirrorOn();
}
void fullyCloseLid(){
while(analogRead(potentiometerPin)>=690){
Serial.println(analogRead(potentiometerPin));
digitalWrite(relay2,LOW);
digitalWrite(relay3,LOW);
}
digitalWrite(relay2,HIGH);
digitalWrite(relay3,HIGH);
}
void fullyOpenLid(){
while(analogRead(potentiometerPin)<=883-10){
Serial.println(analogRead(potentiometerPin));
digitalWrite(relay1,LOW);
digitalWrite(relay4,LOW);
}
digitalWrite(relay1,HIGH);
digitalWrite(relay4,HIGH);
}